The MSP430 Value Line LaunchPad development board is come with the eclipse based Texas Instruments integrated development environment (IDE) called Code Composer Studio (downloadable from the TI website) and equiped with the professional grade C compiler and debugger, which make the development of MSP430 microcontroller based embedded system become easy and fun.
As you know most of the electronics hobbyist used the popular 8-bit class microcontroller to most of their embedded project such as AVR microcontroller from Atmel and PIC microcontroller from Microchip. Now you might wonder why we have to learn another type of microcontroller as most of the modern microcontroller has already provided all the necessary features that we need. Why not, learning another type of microcontroller is one of the fascinating and challenging topics to be learned especially for the true electronics hobbyist as this will broaden our knowledge and utilize what is the best on each of the microcontroller types to support our future embedded system project.
The MSP430 Microcontroller Project
After many considerations of what is the attractive way to introduce this MSP430 microcontroller, instead of just started with a common blinking LED, I decided to built a simple and yet most popular robot,…yes,…is another Line Follower Robot (LFR) using the Texas Instruments 14 pins 16-bit MSP430G2231 microcontroller that come with the MSP430 Value Line LaunchPad development board. Because I think building a robot will give you the basic knowledge and understanding you needs to start explores many of the advance features offered by this 16-bit MSP430 value lines microcontrollers by yourself.
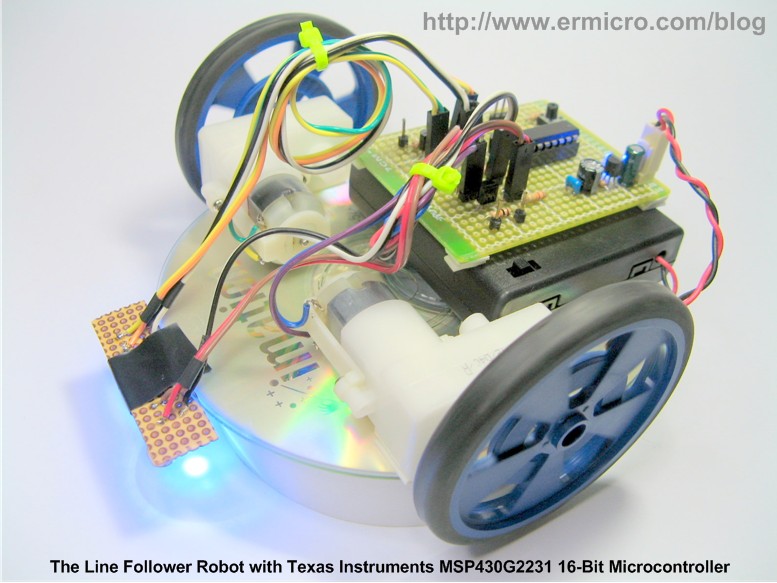
If you notice on the above picture this Line Follower Robot (LFR) used a similar CD chassis, DC geared motor, and sensors found on my previous articles “The LM324 Quad Op-Amp Line Follower Robot with Pulse Width Modulation“. Therefore this project also serves as a good example of the “digital” version of the analog LFR we’ve built before.
The following is the complete electronic schematic of the Line Follower Robot:
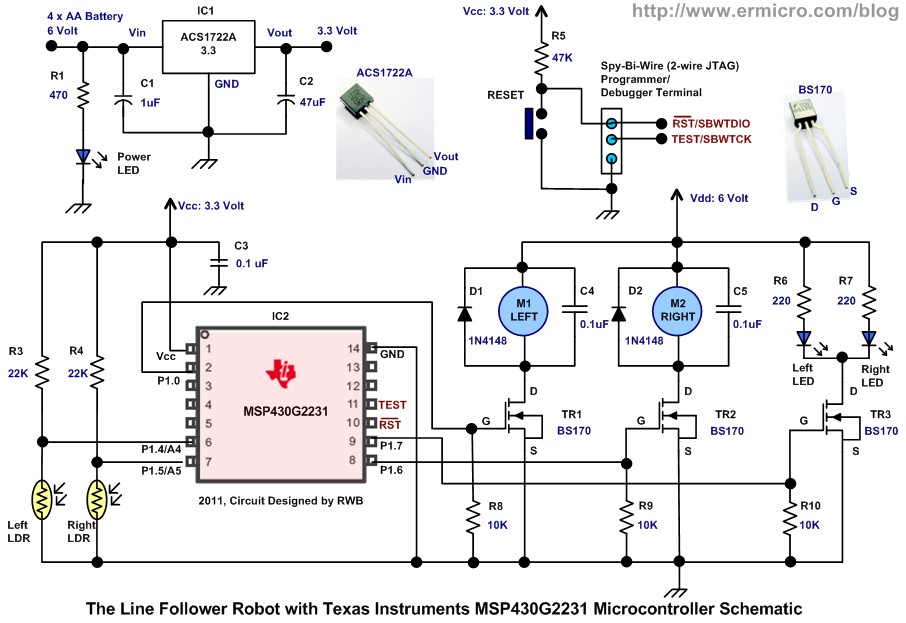
Now let list down all the necessary electronic components and other supported materials to build this LFR:
1. Resistors: 220 (2), 470 (1), 10K (3), 22K (2), and 47K (1)
2. Light Dependent Resistor (2)
3. Capacitors: 0.1uF (3), 1uF (1) and 47uF/16v (1)
4. Diodes: 1N4148 (2)
5. High Intensity 3 mm blue Light Emitting Diode (3)
6. N-Channel MOSFET: BS170 (3)
7. IC: ACS 1722A 3.3 volt voltage regulator or equivalent
8. Texas Instruments MSP430 Value Line LaunchPad Development Board
9. DC Motor: Solarbotics GM2 Geared DC motor with Wheel (2)
10. One reset push button switch
11. Perforated PCB: 70 x 55 mm for the main board and 50 x 15 mm for the sensors board
12. 4 x AA Battery holder
13. CD/DVD ROM (2)
14. Plastic Beads and Paper Clip for the castor (the third wheel)
15. Bolt, Nuts, Double Tape and Standard Electrical Tape for the black line
16. Texas Instruments Code Composer Studio Core Edition version 4.2.1.00004 (used in this project)
17. Texas Instruments MSP430G2231 microcontroller datasheet SLAS694 and SLAU144F.
The complete firmware for this Line Follower Robot project is developed with the C language:
/***************************************************************************** // File Name : LineFollower.c // Version : 1.0 // Description : The MSP430G2231 Line Follower Robot // Author : RWB // Target : MSP430G2231 Custom Line Follower Board // Compiler : Code Composer Studio Version: 4.2.1.00004 // IDE : Code Composer Studio Version: 4.2.1.00004 // Programmer : Texas Instruments MSP430 Launchpad Board // Last Updated : 16 Aug 2011 *****************************************************************************/ #include <msp430g2231.h>
#define LEFT_MOTOR BIT0 #define RIGHT_MOTOR BIT6 #define LEFT_LDR BIT4 #define RIGHT_LDR BIT5 #define SENSOR_LED BIT7
#define MAX_COUNT 100
// Sensor Calibration #define CAL_SAMPLES 5 #define CAL_SPEED1 75 #define CAL_SPEED2 40 #define CAL_MOVE_DELAY 320
// PWM Duty Cycle Threshold #define MAX_THRESHOLD 75 #define MIN_THRESHOLD 60
// Sensor Status #define LEFT_SENSOR 0 #define RIGHT_SENSOR 1
unsigned int pwm_count=0; unsigned int pwm_m1=0; unsigned int pwm_m2=0; unsigned int min_leftLDR=0; unsigned int max_leftLDR=0; unsigned int min_rightLDR=0; unsigned int max_rightLDR=0;
unsigned int adc2cycle(unsigned int adc, unsigned int in_min, unsigned int in_max) { unsigned int adc_val; // Calculate the result and put it within 0 to 100% PWM Duty Cycle value adc_val = 100 - ((adc - in_min) * 100 / (in_max - in_min)); if (adc_val <= MIN_THRESHOLD) adc_val=0; if (adc_val >= MAX_THRESHOLD) adc_val=MAX_THRESHOLD; return(adc_val); }
void DelayMs(unsigned int ms) { while(ms--) { __delay_cycles(1000); // 1 ms delay for 1 MHz Internal Clock } }
// TimerA Channel 0 interrupt service routine #pragma vector=TIMERA0_VECTOR __interrupt void Timer_A (void) { // The PWM Period is about: 101 x 0.1 ms = 10.1 ms pwm_count++; if (pwm_count >= MAX_COUNT) { pwm_count=0; P1OUT |= LEFT_MOTOR; // Turn On Left Motor P1OUT |= RIGHT_MOTOR; // Turn On Right Motor }
if (pwm_count == pwm_m1) { P1OUT &= ~LEFT_MOTOR; // Turn Off Left Motor } if (pwm_count == pwm_m2) { P1OUT &= ~RIGHT_MOTOR; // Turn Off Right Motor } }
unsigned int ReadSensor(unsigned char chn_stat) { ADC10CTL0 &= ~ENC; // Disable ADC10 if (chn_stat) { ADC10CTL1 &= ~INCH_4; // Deselect ADC Channel 4 ADC10CTL1 |= INCH_5; // Select ADC Channel 5 (A5), Right LDR } else { ADC10CTL1 &= ~INCH_5; // Deselect ADC Channel 5 ADC10CTL1 = INCH_4; // Select ADC Channel 4 (A4), Left LDR } ADC10CTL0 |= ENC + ADC10SC; // Enable ADC10 and Conversion start while (ADC10CTL1 & ADC10BUSY); // Wait for ADC Conversion return(ADC10MEM); // Return ADC Value }
void CalibrateSensor() { unsigned char i; unsigned int tmp_left,tmp_right; // Get the Maximum Value Sensor Value (over black line) P1OUT |= SENSOR_LED; // Turn On the Sensor LED DelayMs(1000); // Give enough time to light the LDR tmp_left=0; tmp_right=0; for(i=0; i < CAL_SAMPLES; i++) { tmp_left += ReadSensor(LEFT_SENSOR); // Read The Left LDR (A4) __delay_cycles(50); tmp_right += ReadSensor(RIGHT_SENSOR); // Read The Right LDR (A5) __delay_cycles(50); } max_leftLDR = tmp_left / CAL_SAMPLES; // Get the Max Left Average Value max_rightLDR = tmp_right / CAL_SAMPLES; // Get the Max Right Average Value // Now move the robot to the next calibration stage pwm_m1=CAL_SPEED1; pwm_m2=CAL_SPEED2; DelayMs(CAL_MOVE_DELAY); // Turn off the Motor (Duty Cycle 0) pwm_m1=0; pwm_m2=0; // Get the Minimum Value Sensor Value (over white line) tmp_left=0; tmp_right=0; for(i=0; i < CAL_SAMPLES; i++) { tmp_left += ReadSensor(LEFT_SENSOR); // Read The Left LDR (A4) __delay_cycles(50); tmp_right += ReadSensor(RIGHT_SENSOR); // Read The Right LDR (A5) __delay_cycles(50); } min_leftLDR = tmp_left / CAL_SAMPLES; // Get the Min Left Average Value min_rightLDR = tmp_right / CAL_SAMPLES; // Get the Min Right Average Value // Blink the Sensor LED after calibrating for(i=0; i < CAL_SAMPLES; i++) { P1OUT &= ~SENSOR_LED; // Turn Off LED DelayMs(500); P1OUT |= SENSOR_LED; // Turn On LED DelayMs(30); } }
void main(void) { unsigned int sensor_val; WDTCTL = WDTPW + WDTHOLD; // Stop WDT // P1.0,P1.6 and P1.7 output, Other as Input P1DIR = LEFT_MOTOR + RIGHT_MOTOR + SENSOR_LED;
// Enable the pull-down resistor on the unused input ports P1REN = BIT1 + BIT2 + BIT3; P2REN = BIT6 + BIT7;
// Reset all the Output P1OUT = 0x00; // TIMER A channel 0 will interrupt every 100 cycles // Interrupt time counter period: 100 / 1.000.000 = 0.1 ms TACCTL0 = CCIE; // CCR0 interrupt enabled TACCR0 = 99; TACTL = TASSEL_2 + MC_1; // Start Timer, SMCLK, Up Mode // Start the ADC10 Peripheral // Vref = Vcc, 16 ADC Clock, Enable ADC10 ADC10CTL0 = SREF_0 + ADC10SHT_3 + ADC10ON; // Sample-and-hold ADC10SC bit, ADC10 Clock /1, ADC10 Source Clock, Single Channel Conversion ADC10CTL1 = SHS_0 + ADC10DIV_0 + ADC10SSEL_0 + CONSEQ_0; ADC10AE0 = LEFT_LDR + RIGHT_LDR; // Enable A4 and A5 as ADC Input DelayMs(1); // Wait for ADC Ref to settle // Initial the PWM Duty Cycle and Enable the MSP430 Interrupts pwm_count=0; pwm_m1=0; pwm_m2=0; __enable_interrupt(); // Now we Calibrate the LDR Sensors CalibrateSensor(); DelayMs(1000); // Delay 1000 ms before start // Loop Forever for(;;) { // Read the Left LDR Sensor and make sure is within the range sensor_val=ReadSensor(LEFT_SENSOR); if (sensor_val > max_leftLDR) sensor_val=max_leftLDR; if (sensor_val < min_leftLDR) sensor_val=min_leftLDR; // Assigned the Left PWM Duty Cycle pwm_m1=adc2cycle(sensor_val,min_leftLDR,max_leftLDR); __delay_cycles(20); // Read the Right LDR Sensor and make sure is within the range sensor_val=ReadSensor(RIGHT_SENSOR); if (sensor_val > max_rightLDR) sensor_val=max_rightLDR; if (sensor_val < min_rightLDR) sensor_val=min_rightLDR; // Assigned the Right PWM Duty Cycle pwm_m2=adc2cycle(sensor_val,min_rightLDR,max_rightLDR); __delay_cycles(20); } }
/* EOF: LineFollower.c */The Line Follower Robot Working Principle
This Line Follower Robot design used the photocell sensor known as a Light Dependent Resistor (LDR) made from Cadmium Sulphide (CdS) to detect the black track line, when the LDR is above the black track line it will give a high resistance value while above the white background and it will give a low resistance value. Together with the 22K resistor, they will form what’s known as the voltage divider circuit. This voltage divider circuit sensor will provide the varying voltage according to the amount of the light intensity reflected back to the LDR. The blue Light Emitting Diode (LED) will provide a constant light source for the sensors.
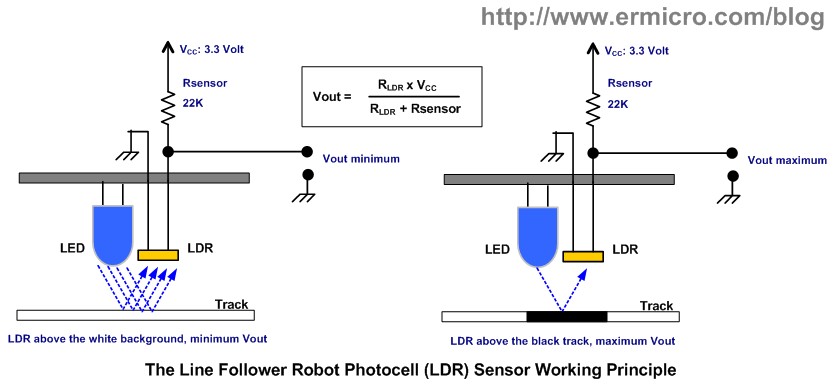
Next the MSP430G2231 microconttroller will translate this varying voltage using its analog to digital conversion (ADC) peripheral into the DC motor rotation speed using what known as the Pulse Width Modulation (PWM) signal. Because this LFR used the “differential steering” (i.e. used two independent DC motor for steering) method, therefore by varying the left and the right DC motor rotation speed proportionally to the light intensity received by both of the left and right LDR, we could easily make the robot to navigate the black track line successfully.
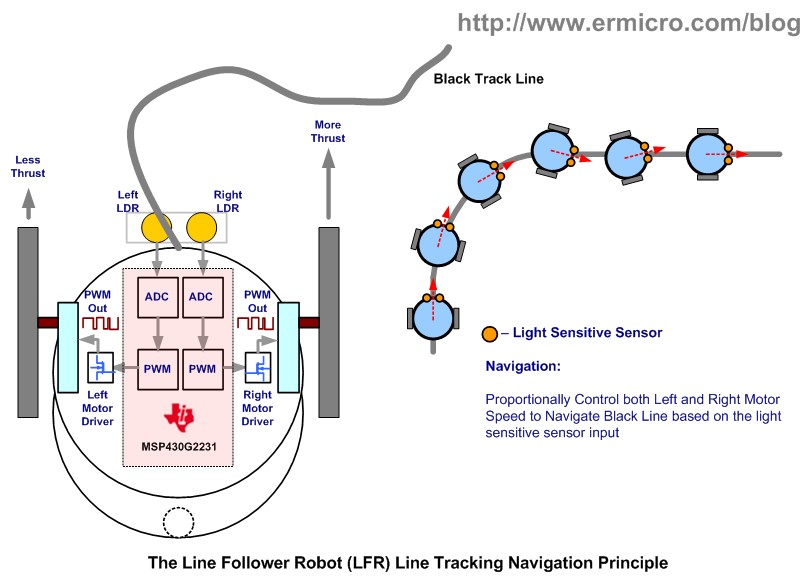
The MSP430G2231 Microcontroller
The Mixed Signal Processing (MSP) 430 series microcontroller is first introduced in the late of 1990 by Texas Instruments. It’s a 16-bit RISC (reduced instruction set computer) microcontroller with Von Neumann architecture where the CPU, I/O, and memory shared the same 16-bit control, address, and data bus. The MSP430 is specially design for low consumption and optimize to be used with the C compiler.
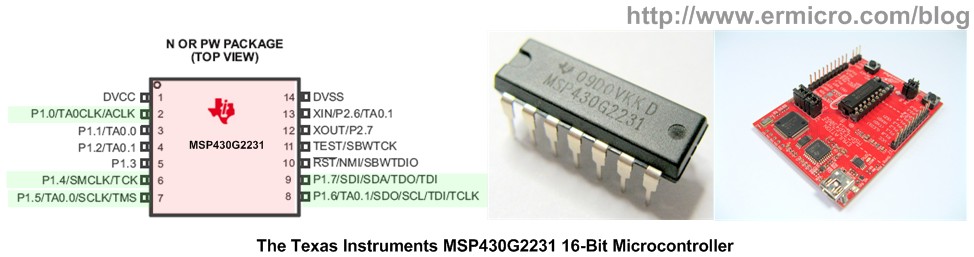
The “G” value line series such as the 14-pin MSP430G2231 microcontroller is introduced together with the price phenomenal LaunchPad development board. This microcontroller has these following interesting features which I’m sure as the electronics hobbyist you will eager to try it by yourself.
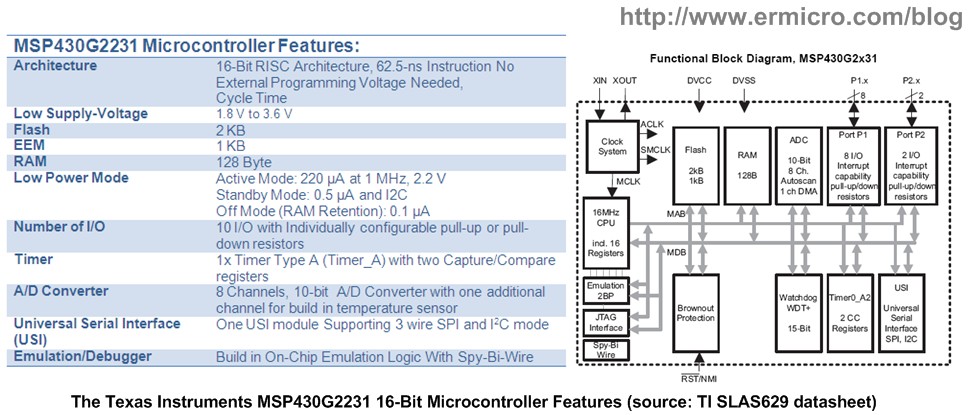
One of the features that make this 14-pin MSP430G2231 microcontroller special is the build in on-chip emulation logic using what is called “Spy-Bi-Wire” or also known as 2-wire JTAG (Joint Test Action Group). This useful feature enables us to step the C code line by line, set a break point, and check the variables or registers value while the chip is in the circuit (in circuit programming and debugging).
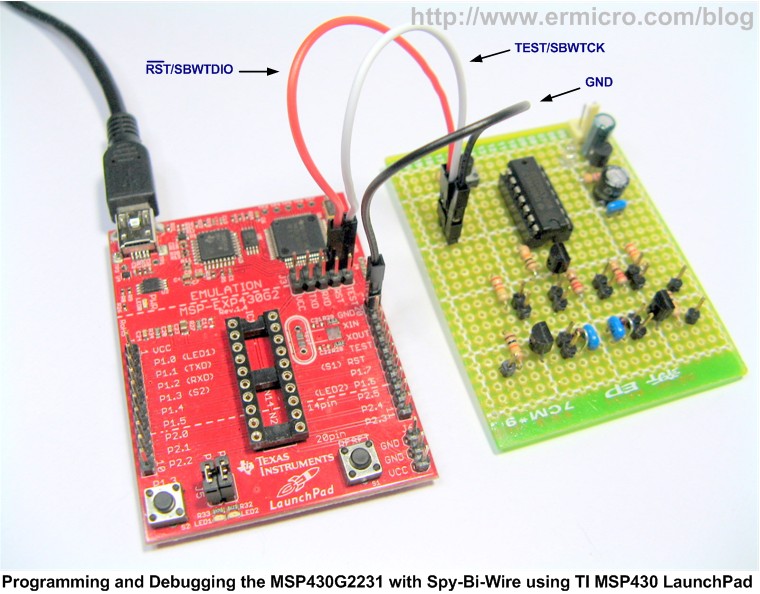
The MSP430G2231 Microcontroller Input/Output (I/O)
The MSP430G2231 microcontroller has 10 I/O, 8 pins on the first ports (P1) and 2 pins on the second ports (P2). All these ports are configurable as the general purpose input or output ports and often they multiplexed with other I/O function such as A/D (analog to digital) input, PWM out, USI (Universal Serial Interface), Clock Input, Crystal Oscillator Input, and JTAG I/O terminal.
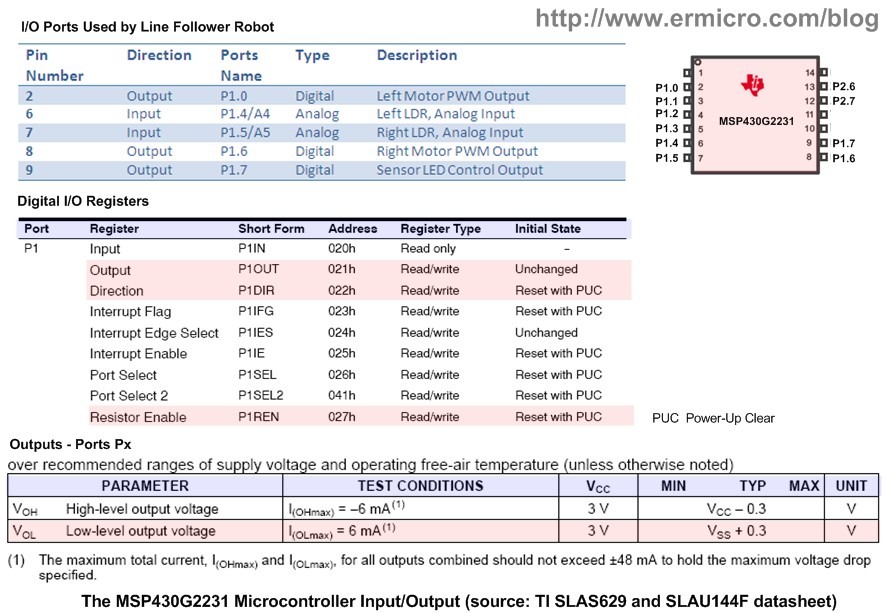
As you’ve seen from the table above, the LFR only used several I/O ports and as rules of thumb the unused I/O ports have to be configured as the output ports and leave them unconnected. Alternatively you could configure all the unused ports as the input ports (default on power-up reset) and enable the pull-down resistor in order to avoid the unpredictable “floating” inputs problem arise in your project. The following C code shows how to configure the necessary I/O ports for this LFR:
#define LEFT_MOTOR BIT0 #define RIGHT_MOTOR BIT6 #define LEFT_LDR BIT4 #define RIGHT_LDR BIT5 #define SENSOR_LED BIT7 ...
// P1.0,P1.6 and P1.7 output, Other as Input P1DIR = LEFT_MOTOR + RIGHT_MOTOR + SENSOR_LED;
// Enable the pull-down resistor on the unused input ports P1REN = BIT1 + BIT2 + BIT3; P2REN = BIT6 + BIT7;
// Reset the Output P1OUT = 0x00;The P1DIR (port 1 direction) register is used to configure the I/O port direction, where each bit of this 16-bit corresponding to the I/O ports (P1.0 to P1.7). By enabling the corresponding bit we simply tell the MSP430G2231 microcontroller to configure the port as an output port. Next the P1REN (port 1 pull-up/pull-down resistor) register, by enabling the corresponding bit we could enable the pull-down resistor (configured as input) or pull-up resistor (configured as output).
The MSP430G2231 microcontroller P1OUT (port 1 output) register is used to control the output port logical state, it used to turn on and off the P1.0 and P1.6 to generate the required PWM signal. I used these ports because these ports are connected with two LED in the MSP430 LaunchPad development board, therefore you could easily test the PWM output using these LEDs. The P1.7 output port is also used to control the sensor LED; beside as the sensor light source, it also serves as a sign indicator when the LFR finish calibrating the sensors. The following code use C language bit operator to turn on and off the port using the MSP430G2231 microcontroller P1OUT register:
// Reset all the Output P1OUT = 0x00; ... ... P1OUT |= LEFT_MOTOR; // Turn On Left Motor P1OUT |= RIGHT_MOTOR; // Turn On Right Motor ... ... P1OUT &= ~LEFT_MOTOR; // Turn Off Left Motor ... ... P1OUT &= ~RIGHT_MOTOR; // Turn Off Right Motor ... ... P1OUT &= ~SENSOR_LED; // Turn Off LED ... P1OUT |= SENSOR_LED; // Turn On LED ... ...From the data sheet the maximum output current for each port is about 6 mA and for all outputs combined is about 48 mA, this of course is not suitable for driving the DC motor directly; therefore in this project I used the n-channel MOSFET (Metal Oxide Semiconductor Field Effect Transistor) BS170 to drive the DC motor and sensor LED. The advantage of using MOSFET because this type of transistor has very high input impedance on its Gate (G) terminal which mean its need very low current in order to operate and its has a low ON resistance between the its Drain (D) and Source (S) terminals called Rds especially when operate on higher DC voltage supply compare to the ordinary Bipolar Junction Transistor (BJT).
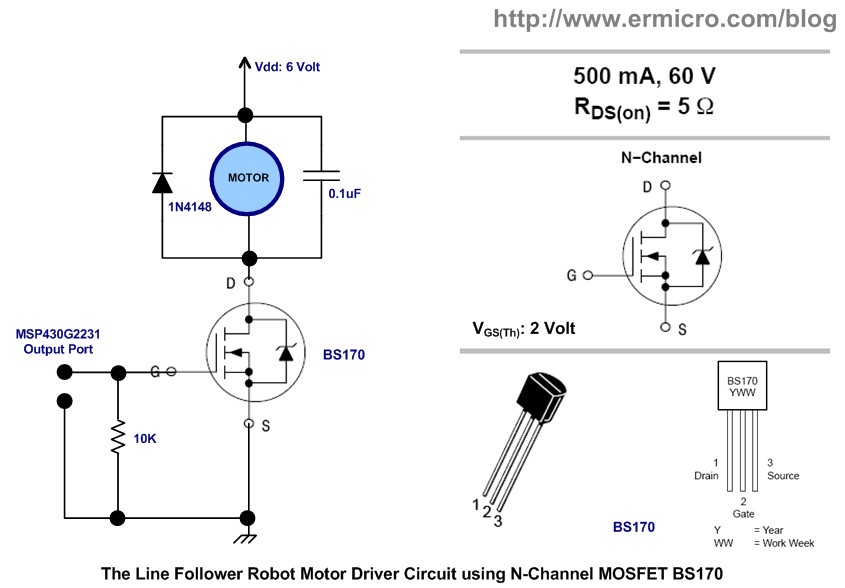
By applying voltage greater than the Vgs threshold voltage i.e. voltage applied between the Gate and Source terminal, it’s about 2 volt on the BS170 MOSFET, we could bring the MOSFET into its saturate stage (ON) and this voltage level could be easily provided by the MSP430G2231 microcontroller output port.
The MSP430G2231 Pulse Width Modulation
Pulse Width Modulation (PWM) is a technique widely used in modern switching circuit to control the amount of power given to the electrical device (i.e. the DC motor). By simply switches ON and OFF the power supplied to the DC motor rapidly and the average amount of energy received by the DC motor is corresponding to the ON and OFF period (duty cycle); therefore by varying the ON period i.e. longer or shorter than the OFF period, we could control the DC motor rotation speed.
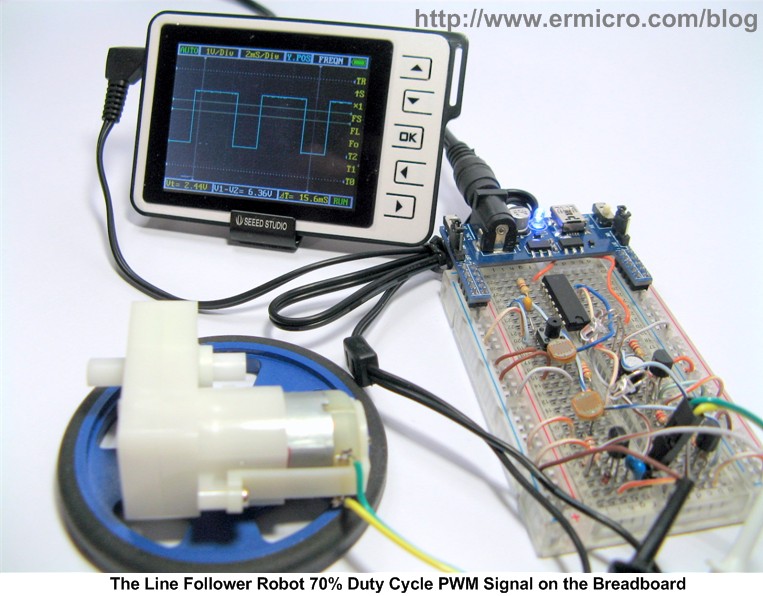
The MSP430G2231 microcontroller actually has two capture/compare registers that could be used for generating the PWM automatically, but because we need two independent PWM sources with the configurable PWM duty cycle and on the specific PWM frequency, therefore we could not use the built in PWM which is provided by the MSP4302231 microcontroller. Instead on this LFR project I used the software PWM which is based on the MSP430G2231 Timer_A channel 0 interrupt.
The basic software PWM could be made by first creating the basic digital ramp counter for the PWM signal period and then use the variable to be compared with the ramp counter value and this will create the necessary PWM duty cycle as shown on this following diagram:
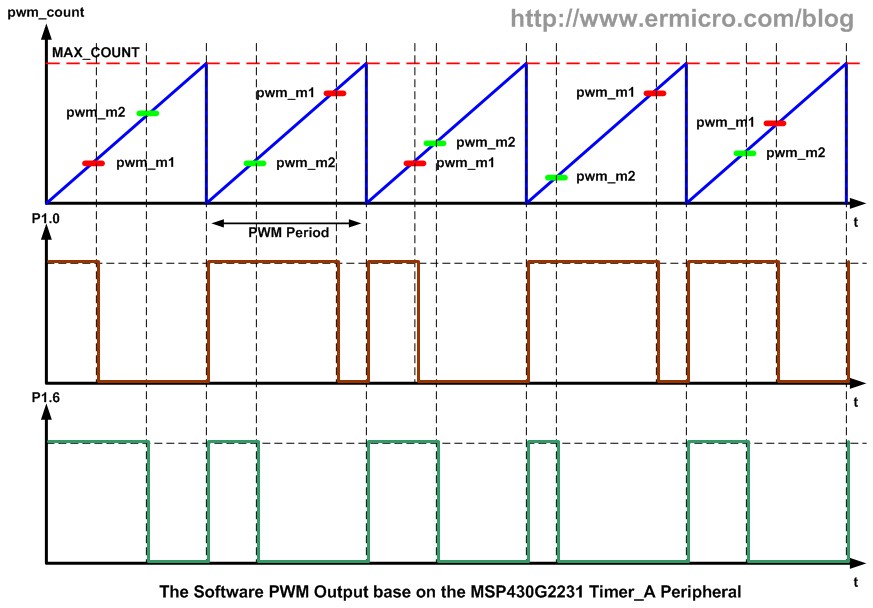
The basic digital ramp counter used the pwm_count variable to count from 0 to MAX_COUNT and start to count from 0 again repeatedly. The pwm_count will provide a constant period to the PWM signal. Next we need two variables pwm_m1 and pwm_m2 to be compared with the pwm_count variable. When the pwm_count reach 0, we simply turn ON the MSP430G2231 microcontroller output port and when the pwm_count equal to the pwm_m1 or pwm_m2 value, we simply turn OFF the MSP430G2231 microcontroller output port. Therefore by varying both of the pwm_m1 or pwm_m2 variables value we could control the PWM signal duty cycle.
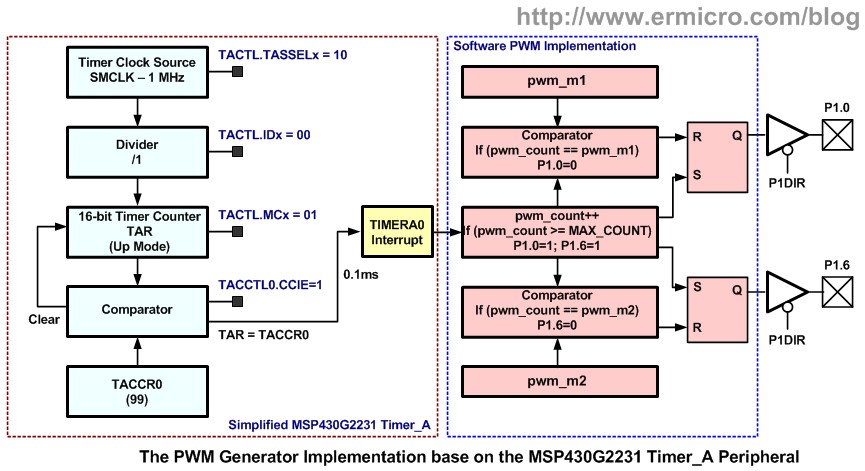
We used the MSP430G2231 microcontroller Timer_A in “Up Mode” to actually increase and control the pwm_count value and when the Timer_A counter register (TAR) equal to Timer A Capture/Control Register channel 0 (TACCR0) it will generate the interrupt. Because on this project I used MSP430G2231 microcontroller standard Sub Main Clock (SMCLK) of 1 MHz for the Timer_A clock source, thus assigning 99 to the TACCR0 register will make the Timer_A channel 0 to generate interrupt on every 100 (TACCR0 + 1) cycles or about 0.1 ms as shown on this following C code:
// TIMER A channel 0 will interrupt every 100 cycles // Interrupt time counter period: 100 / 1.000.000 = 0.1 ms TACCTL0 = CCIE; // CCR0 interrupt enabled TACCR0 = 99; TACTL = TASSEL_2 + MC_1; // Start Timer, SMCLK, Up ModeThe software PWM implementation is implemented inside the Timer_A channel 0 interrupt function handler as show on this following C code:
// TimerA Channel 0 interrupt service routine #pragma vector=TIMERA0_VECTOR __interrupt void Timer_A (void) { // The PWM Period is about: 101 x 0.1 ms = 10.1 ms pwm_count++; if (pwm_count >= MAX_COUNT) { pwm_count=0; P1OUT |= LEFT_MOTOR; // Turn On Left Motor P1OUT |= RIGHT_MOTOR; // Turn On Right Motor }
if (pwm_count == pwm_m1) { P1OUT &= ~LEFT_MOTOR; // Turn Off Left Motor } if (pwm_count == pwm_m2) { P1OUT &= ~RIGHT_MOTOR; // Turn Off Right Motor } }By choosing MAX_COUNT of 100, we could get the PWM period about 101 x 0.1ms, which is about 10.1 ms or we could say that the PWM frequency is about 100 Hz and by assigning each of the pwm_m1 and pwm_m2 variables value from 0 to 100, we could get the PWM duty cycle output varying from 0 to 100%.
The pwm_m1 and pwm_m2 variables value is supplied by the digital value from the left and the right sensors from the adc2cycle() function which basically set the upper and lower PWM duty cycle value returned to these variables. The upper and lower threshold setting is depend on the black line track and the sensors characteristic and could be changed by changing each of the MAX_THRESHOLD and the MIN_THRESHOLD definition value.
The MSP430G2231 ADC Peripheral
The MSP430G2231 microcontroller has one 10-bit Analog to Digital Conversion (ADC) peripheral also known as ADC10 peripheral with 8 channel (A0 to A7), where the channel (A10) is specially used for the internal thermometer. The MSP430G2231 ADC10 peripheral used what is called “Successive Approximation Method” to convert the analog input from one of these channels to the 10-bit digital representation and stores the result in the ADC10MEM register.
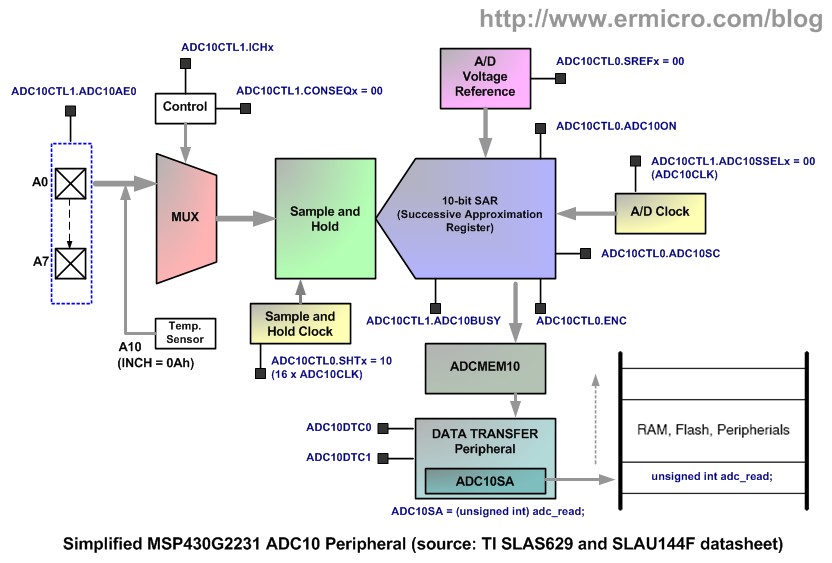
The ADC10 peripheral is controlled by two control registers, ADC10CTL0 and ADC10CTL1. Thus by setting the ADC10ON bit (logical high) in ADC10CTL0 register we enable this ADC core. The most important thing to remember that these ADC10 control registers can only be modified when ENC (Enable Conversion) bit in ADC10CTL0 is low (ENC = 0) and prior to the A/D conversion this bit has to be set to 1 (logical high).
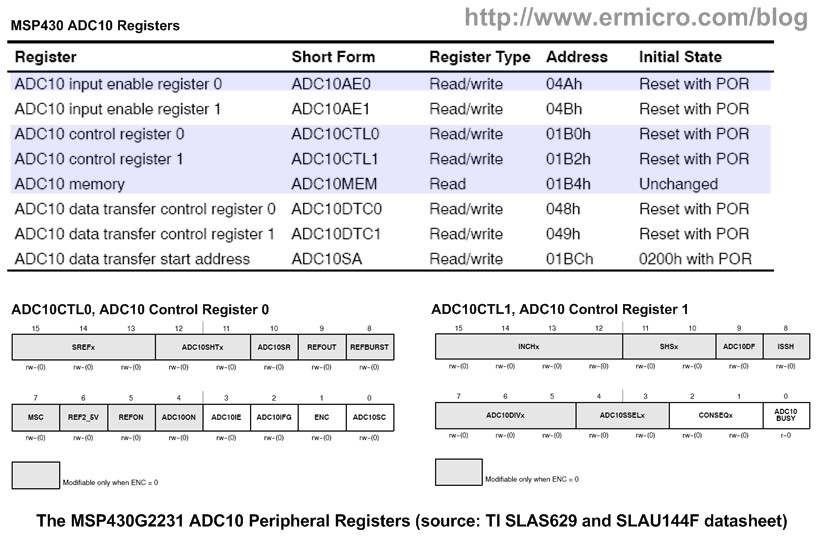
The MSP430G2231 ADC10 peripheral have four operating mode which could be selected by setting the CONSEQx bits in the ADC10CTL1 (ADC10 Control Register 1) and on this LFR project we will use the “Single Channel Single Conversion Mode“. The following C code show how we setup the MSP430G2231 microcontroller ADC10 peripheral:
// Start the ADC10 Peripheral // Vref = Vcc, 16 ADC Clock, Enable ADC10 ADC10CTL0 = SREF_0 + ADC10SHT_3 + ADC10ON; // Sample-and-hold ADC10SC bit, ADC10 Clock /1, ADC10 Source Clock, Single Channel Conversion ADC10CTL1 = SHS_0 + ADC10DIV_0 + ADC10SSEL_0 + CONSEQ_0; ADC10AE0 = LEFT_LDR + RIGHT_LDR; // Enable A4 and A5 as ADC Input DelayMs(1); // Wait for ADC Ref to settleThe multiplexer analog channels input could be selected by assigning the corresponding INCHx bits in the ADC10CTL1 register. The actual A/D conversion is take placed in the ReadSensor() function, as shown on this following C code:
unsigned int ReadSensor(unsigned char chn_stat) { ADC10CTL0 &= ~ENC; // Disable ADC10 if (chn_stat) { ADC10CTL1 &= ~INCH_4; // Deselect ADC Channel 4 ADC10CTL1 |= INCH_5; // Select ADC Channel 5 (A5), Right LDR } else { ADC10CTL1 &= ~INCH_5; // Deselect ADC Channel 5 ADC10CTL1 = INCH_4; // Select ADC Channel 4 (A4), Left LDR } ADC10CTL0 |= ENC + ADC10SC; // Enable ADC10 and Conversion start while (ADC10CTL1 & ADC10BUSY); // Wait for ADC Conversion return(ADC10MEM); // Return ADC Value }Noticed on the C code above that before we change the ADC10 control register (i.e. ADC10CTL0 and ADC10CTL1), we have to disable the ADC10 first be resetting the ENC bit on ADC10CTL0 register then prior to the A/D conversion we set (enable) the ENC and ADC10SC (ADC10 Start Conversion) bits in ADC10CTL0 register. Next we wait the conversion by checking the ADC10BUSY bit on the ADC10CTL1 register. When the ADC10BUSY bit is become “0″ means the conversion is done and we could retrieve the stored 10-bit digital value in the ADC10MEM register.
One of the most important features on this LFR project is the used of the calibration phase in the CalibrateSensor() function. In the calibration phase we read the sensors for their maximum value (i.e. on the black line) and the minimum (i.e. on the white background) value. This calibration phase will ensure both of the left and right sensors provide equal value to the PWM generator for driving DC motor. The actual algorithm to make this LFR navigate the black track line successfully is shown on this following C code:
// Loop Forever for(;;) { // Read the Left LDR Sensor and make sure is within the range sensor_val=ReadSensor(LEFT_SENSOR); if (sensor_val > max_leftLDR) sensor_val=max_leftLDR; if (sensor_val < min_leftLDR) sensor_val=min_leftLDR; // Assigned the Left PWM Duty Cycle pwm_m1=adc2cycle(sensor_val,min_leftLDR,max_leftLDR); __delay_cycles(20); // Read the Right LDR Sensor and make sure is within the range sensor_val=ReadSensor(RIGHT_SENSOR); if (sensor_val > max_rightLDR) sensor_val=max_rightLDR; if (sensor_val < min_rightLDR) sensor_val=min_rightLDR; // Assigned the Right PWM Duty Cycle pwm_m2=adc2cycle(sensor_val,min_rightLDR,max_rightLDR); __delay_cycles(20); }The Line Follower Robot Assembly
The Line Follower Robot first is constructed on the breadboard in order to test the circuit before I move it to the perforated PCB (70 x 55 mm); I used a similar wiring method to wire the circuit on the main LFR perforated PCB as explained on my previous article “Quick and Efficiently Wiring Your Prototype Circuit Board“.
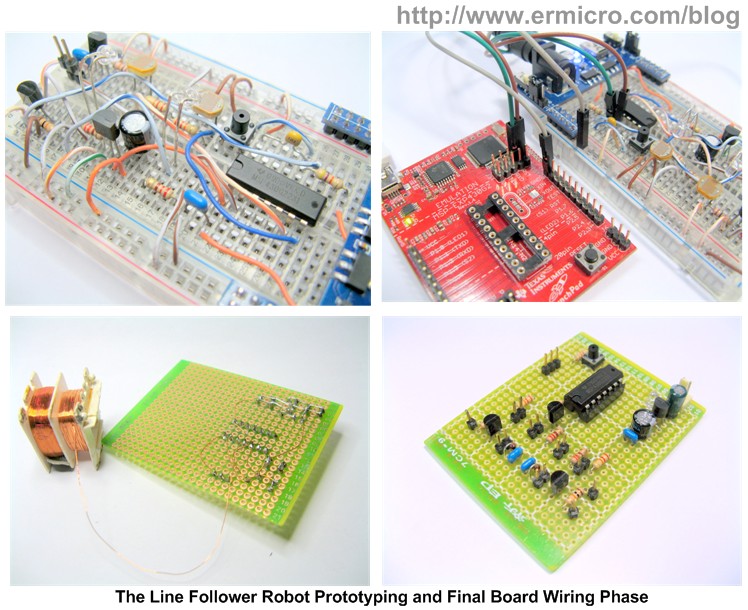
The Line Follower Robot construction could be constructed freely but the easiest one is to use the discarded CD/DVD ROM as shown on these following pictures:
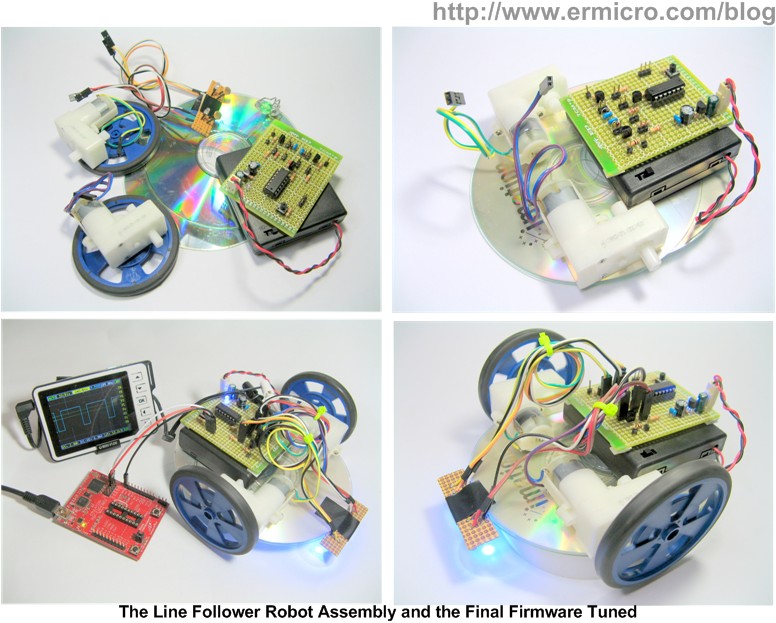
I glued the two CDROM together in order to make more room and attached the two GM2 DC motors, 4xAA battery holder, main board, and sensor board using the double tape. The sensors (LDR and LED) are constructed in a small perforated PCB (50 x 15 mm) with this following guidance:
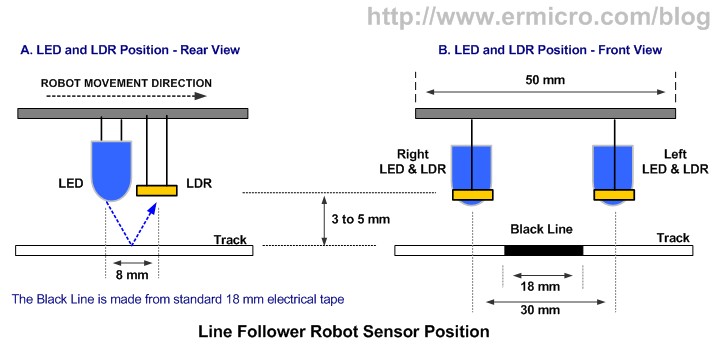
Finally using the Texas Instruments MSP430 Value Line LaunchPad development board Spy-Bi-Wire connector and the Texas Instruments Code Composer Studio Core Edition v4.2.1.00004 (used in this project), we could easily programming and debugging the LFR firmware:
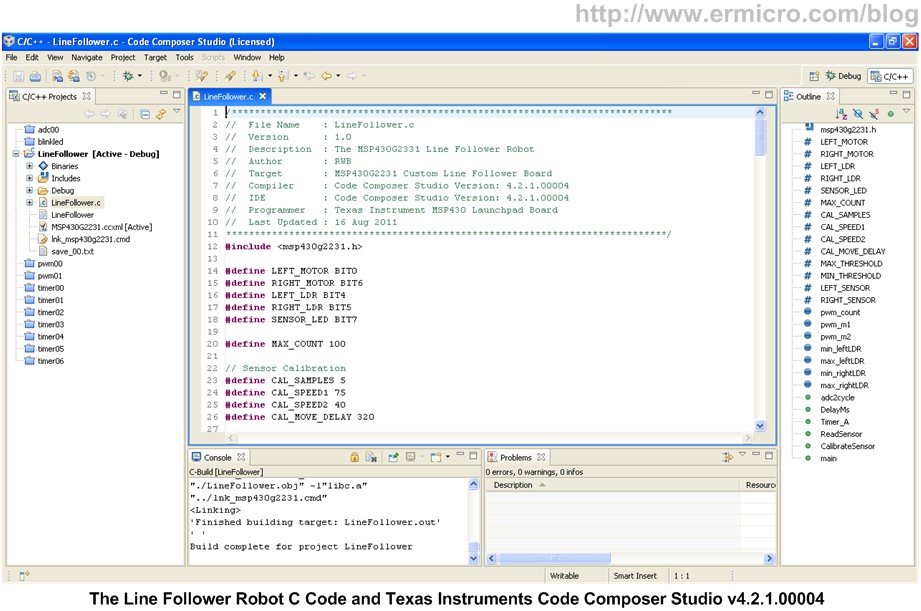
4 komentar:
Thanks for all the guidelines. This details will be really beneficial in my look for.
you are welcome :)
wis gek ndang di realisasikan, hehehe
monggo mas danang........
Post a Comment